Open Source
TypeScript AI AgentFramework
Escape no-code limits and scratch-built overhead. Build, customize, and orchestrate AI agents with full control, speed, and a great DevEx.
DEVELOPMENT & ORCHESTRATION
Build agents with VoltAgentVoltAgent Framework
open-sourceA TypeScript framework for building and AI agents with enterprise-grade capabilities and seamless integrations.
LLM OBSERVABILITY PLATFORM
Gain visibility with VoltOpsVoltOps LLM Observability
Framework-agnostic observability platform for tracing, debugging, and monitoring AI agents across tech stacks.
import { VoltAgent, Agent, createTool, createHooks } from "@voltagent/core";
import { VercelAIProvider } from "@voltagent/vercel-ai";
import { openai } from "@ai-sdk/openai";
import { fetchRepoContributorsTool } from "./tools";
import { fetchRepoStarsTool } from "./tools";
// Create the stars fetcher agent
const starsFetcherAgent = new Agent({
name: "Stars Fetcher",
description: "Fetches the number of stars for a GitHub repository using the GitHub API",
llm: new VercelAIProvider(),
model: openai("gpt-4o-mini"),
tools: [fetchRepoStarsTool],
});
// Create the contributors fetcher agent
const contributorsFetcherAgent = new Agent({
name: "Contributors Fetcher",
description: "Fetches the list of contributors for a GitHub repository using the GitHub API",
llm: new VercelAIProvider(),
model: openai("gpt-4o-mini"),
tools: [fetchRepoContributorsTool],
});
// Create the analyzer agent
const analyzerAgent = new Agent({
name: "Repo Analyzer",
description: "Analyzes repository statistics and provides insights",
llm: new VercelAIProvider(),
model: openai("gpt-4o-mini"),
});
// Create the supervisor agent that coordinates all the sub-agents
const supervisorAgent = new Agent({
name: "Supervisor",
description: `You are a GitHub repository analyzer. When given a GitHub repository URL or owner/repo format, you will:
1. Use the StarsFetcher agent to get the repository's star count
2. Use the ContributorsFetcher agent to get the repository's contributors
3. Use the RepoAnalyzer agent to analyze this data and provide insights
Example input: https://github.com/voltagent/voltagent or voltagent/voltagent
`,
llm: new VercelAIProvider(),
model: openai("gpt-4o-mini"),
subAgents: [starsFetcherAgent, contributorsFetcherAgent, analyzerAgent],
});
// Initialize the VoltAgent with the agent hierarchy
new VoltAgent({
agents: {
supervisorAgent,
},
});
Read-onlyEnterprise-level AI agents
Complete toolkit for enterprise level AI agents
Design production-ready agents with unified APIs, tools, and memory.
123456789101112131415161718import { Agent } from '@voltagent/core'
import { VercelAIProvider } from '@voltagent/vercel-ai'
import { openai } from '@ai-sdk/openai'
import { anthropic } from '@ai-sdk/anthropic'
const agent = new Agent({
provider: new VercelAIProvider(),
model: openai("gpt-4o-mini"),
});
const anthropicAgent = new Agent({
provider: new VercelAIProvider(),
model: anthropic('claude-3-haiku-20240307'),
});
Enable agents to invoke functions, interact with systems, and perform actions.
Seamlessly switch between different AI providers with a simple code update.
Experiment, fine-tune, and iterate your AI prompts in an integrated environment.
Store and recall interactions to enhance your agents intelligence and context.
Intelligent Coordination
Supervisor agent orchestration
Build powerful multi-agent systems with a central Supervisor Agent that coordinates specialized agents.
12345678910111213141516171819202122232425262728293031323334import { Agent } from "@voltagent/core";
import { VercelAIProvider } from "@voltagent/vercel-ai";
import { openai } from "@ai-sdk/openai";
// Define supervisor agent
const supervisorAgent = new Agent({
name: "Supervisor Agent",
description: "You manage a workflow between specialized agents.",
llm: new VercelAIProvider(),
model: openai("gpt-4o-mini"),
subAgents: [storyAgent, translatorAgent]
});
// Define story agent
const storyAgent = new Agent({
name: "Story Agent",
description: "You are a creative story writer.",
llm: new VercelAIProvider(),
model: openai("gpt-4o-mini"),
});
// Define translator agent
const translatorAgent = new Agent({
name: "Translator Agent",
description: "Translate English text to German",
llm: new VercelAIProvider(),
model: openai("gpt-4o-mini"),
});
// Stream response from supervisor agent
const result = await supervisorAgent.streamText(
"Write a 100 word story in English."
);
for await (const chunk of result.textStream) {
console.log(chunk);
}
RAG
Accurate and context-aware responses
For advanced querying and dynamic analysis, integrate data into a knowledge base by syncing from diverse sources
INTEGRATIONS
Easily connect with 40+ apps in no time
Integrate your AI agents with your preferred tools and services effortlessly.
Stay in control at every stage
From tracking deployments to debugging and live interaction, VoltAgent gives you full visibility into your AI agents.
Deploy your Agents in seconds with VoltAgent Deployment.
Debug and analyze your VoltAgent-powered AI agent's behavior with visual flows.
Connect your VoltAgent-powered AI agents to popular observability platforms.
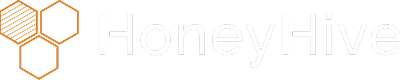

123456789101112131415161718import { VoltAgent } from "@voltagent/core"
import { LangfuseExporter } from "langfuse-vercel"
export const volt = new VoltAgent({
telemetry: {
serviceName: "ai",
enabled: true,
export: {
type: "custom",
exporter: new LangfuseExporter({
publicKey: process.env.LANGFUSE_PUBLIC_KEY,
secretKey: process.env.LANGFUSE_SECRET_KEY,
baseUrl: process.env.LANGFUSE_BASEURL,
}),
},
},
});
Interact with your AI agent through natural language chat interface.
Agent, analyze last month's social media campaign performance and provide recommendations for improvement.
Analysis complete. Last month's campaign reached 45% more users but had 12% lower conversion rate compared to previous campaigns. Key findings: 1) Video content performed 3x better than static images. 2) Posts published between 6-8pm had highest engagement. 3) Product demonstration posts generated most conversions. Recommendation: Increase video content by 40%, focus on product demonstrations, and schedule more posts during evening hours.
Create a content calendar for next month based on these insights. Include optimal posting times and content types.
Content calendar created. I've scheduled 15 posts across platforms with 60% video content focused on product demos. Primary posting times are Tuesdays and Thursdays 6-8pm, with additional posts Monday and Friday mornings based on secondary engagement peaks. I've included hooks for upcoming product launch and integrated seasonal marketing themes. Calendar has been added to your Marketing Projects workspace and synced with team collaboration tools.
Blog
All about AI Agents
We are sharing our knowledge about AI Agents.
GitHub Repo Analyzer Agent: From Zero to...
Build a practical AI agent that analyzes GitHub repositories, identifies...
Escape the 'console.log': VoltOps LLM Observability
Stop drowning in console logs. VoltOps LLM Observability offers unprecedented...
VoltAgent v0.1: AI Development Reimagined for JavaScript/TypeScript
VoltAgent is here! Build, debug, and deploy AI agents with...
Community
Join the movement
Our growing open source community building the future of AI agents.